In programming, in some situation, we might want a certain block of code to be executed. In another situation, we might want to execute another block of code ignoring the earlier block. This is exactly what decision making statements do.
For example, consider you are driving a car. If the traffic signal is red, we should stop. Otherwise you should proceed.
So, we have a condition and a corresponding action. Based on the outcome of the condition, java decides whether to execute a block or not.
If (traffic signal is red)
stop
else
proceed
In java, there are two types of decision-making statements, if-else statement and switch statement.
if statement
As explained above, the if
statement is used to evaluate a condition. This condition must return a boolean value (true or false). If the condition is true, the corresponding block of code is executed.
Syntax:
if (condition) {
// code to be executed if the condition is true
}
Example:
int val = 30;
if (val == 20) {
System.out.println("First block is executed"); ⇐ This won’t be executed
}
if (val != 20) {
System.out.println("Second block is executed"); ⇐ This will be executed
}
Output: Second block is executed
else statement
Along with if
statement, we can also use else
statement to specify a block of code that should be executed if the condition of the if
statement is false.
Syntax:
if (condition) {
// code to be executed if the condition is true
} else {
// code to be executed if the condition is false
}
Example:
int val = 30;
if (val == 20) {
System.out.println("First block is executed");
} else {
System.out.println("Second block is executed");
}
Output: Second block is executed
else if statement
We can also use one or more else if
statements after if
statement to specify conditions if the first if
condition is false.
Syntax:
if (condition1) {
// code to be executed if condition1 is true
} else if (condition2) {
// code to be executed if the condition1 is false and condition2 is true
} else {
// block of code to be executed if both condition1 and condition2 is false
}
Example:
int val = 30;
if (val == 20) {
System.out.println("First block is executed");
} else if (val == 30) {
System.out.println("Second block is executed");
} else {
System.out.println("Third block is executed");
}
Output: Second block is executed
Ternary Operator
There is also a shorthand to write an if-else statement which is called the ternary operator. Let’s understand with an example –
int val = 30;
String result = null;
if (val == 20) {
result = "First block";
} else {
result = "Second block";
}
System.out.println(result);
Output: Second block
We can do the same thing using the ternary operator. The syntax is below –
result = (condition) ? valueIfTrue : valueIfFalse;
Example:
int val = 30;
String result = (val == 20) ? "First block" : "Second block";
System.out.println(result);
Output: Second block
Switch Statement
A switch statement allows a value to be tested against a list of values (cases). The value is checked against each case sequentially and whatever case is matched, the corresponding block of code is executed.
We can have any number of case statements within a switch. A switch statement can also have an optional default
case at the very end of the switch. The default case will be executed when none of the cases are true.
Syntax:
switch(expression) {
case value1:
// code block 1
break;
case value2:
// code block 2
break;
default:
// code block 3
}
Flow Diagram:
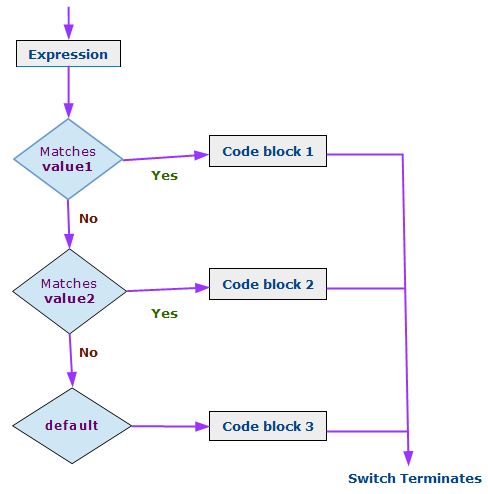
Example:
int value = 20;
switch (value) {
case 10:
System.out.println("Value is 10");
break;
case 20:
System.out.println("Value is 20");
break;
default:
System.out.println("Value is neither 10 or 20");
}
Output: Value is 20
You might wonder why we are using the break
keyword. The break keyword is used to break out of the switch block. For each case, we have a break statement. Because when a match is found, just get out of the switch block. There is no need to check anything else.
Also, if you don’t use the break statement, you might see unexpected results. Let’s remove the break statement and execute the same code.
int value = 20;
switch (value) {
case 10:
System.out.println("Value is 10");
case 20:
System.out.println("Value is 20");
default:
System.out.println("Value is neither 10 or 20");
}
Output:
Value is 20
Value is neither 10 or 20
Explanation:
- Case 10 is false. So execution will go to the next case.
- Case 20 is true. So “Value is 20” is printed. As there is no break statement, execution will go to the next case.
- As the default case doesn’t contain any condition, the corresponding block will be executed and “”Value is neither 10 or 20″ will be printed.
So you see, break doesn’t only skip unnecessary checking, it may also lead to some unexpected result. So always use break statements for each case. For the default case, break is not needed as that is the very last case.
This is pretty much all about decision making statements. We will meet again with a new topic.
Its like you read my mind! You seem to know a lot about this, like you wrote the book in it or something.
I think that you can do with a few pics to drive the message home a bit, but other than that, this
is excellent blog. An excellent read. I will certainly be back.
I am glad you liked it and many thanks for your suggestion 🙂