Wrapper Classes
We all know, there are 8 primitive
data types supported by java. Sometimes you need to treat those primitives as objects (we will discuss why in a moment). For that reason, java provides wrapper classes
for each primitive type that can wrap the primitive data inside an object.
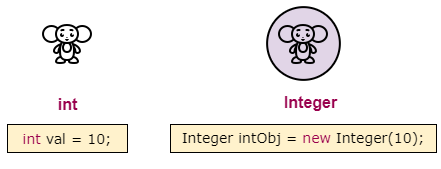
The table below shows the primitive type
and the corresponding wrapper class
–
Primitive Data Type | Wrapper Class |
---|---|
byte | Byte |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
boolean | Boolean |
char | Character |
The table below shows the methods used to get value from wrapper objects.
Primitive Data Type | Method to get primitive value from wrapper Object |
---|---|
byte | byteValue() |
short | shortValue() |
int | intValue() |
long | longValue() |
float | floatValue() |
double | doubleValue() |
boolean | booleanValue() |
char | charValue() |
Let’s try to create an Integer
object and then get the int
value from that object.
Integer wrapperObj = new Integer(10);
int intVal = wrapperObj.intValue();
The sad story before Java 5
Let’s assume you are using the java version prior to 5.0 and you are trying to add an int
value into an ArrayList
.
int val = 10;
List intList = new ArrayList();
intList.add(val);
You will get a compilation error at the third line as the list (or any other collection) is supposed to contain objects, not primitive types.
So, you have to wrap the int
value in an Integer
and only after that the list will allow you to add that object to the list.
int val = 10;
List intList = new ArrayList();
intList.add(new Integer(val));
Now, if you want to get the value from the list, it will come out as an Object
. You have to cast it to Integer
first and then you have to call intValue()
method to convert that Integer
object to int
.
Object obj = intList.get(0);
Integer intObj = (Integer) obj;
int output = intObj.intValue();
As you can see, just to add an int
value to a list and get that value out of that list, we are writing multiple lines of code. This is definitely not looking clean.
The happy ending
As you have seen above, we are manually converting primitive values to wrapper objects and vice versa. To get rid of that irritating task, Java 5.0 introduced new features called autoboxing
and unboxing
to do that conversion automatically.
A brief introduction to Generics
Before Java 5.0, all the collections (like List, Set etc.) were supposed to hold type Object
. So, you can put anything to the collection, but when you get something out of that collection, you will get a reference of type Object
.
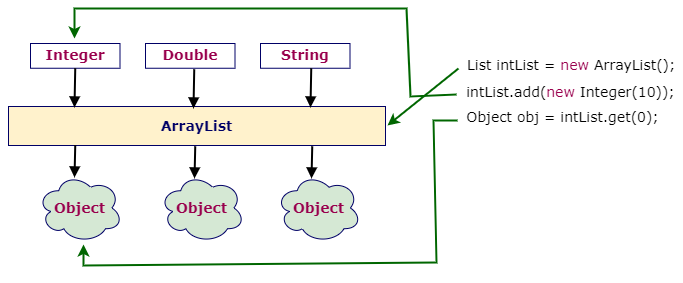
Java 5.0 introduced generics
using which you can create a collection of specific types. That collection will allow you to add elements of that type only and when you get something out of the list, you will get an object of that type only.
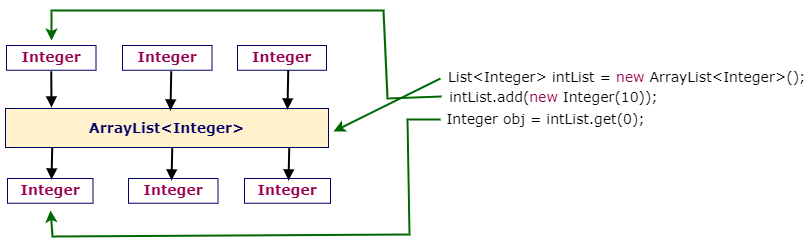
Rewrite the code using generics
int val = 10;
List<Integer> intList = new ArrayList<Integer>();
intList.add(new Integer(val));
Integer intObj = intList.get(0);
int output = intObj.intValue();
This is a little better than the initial version. But we are not done yet.
Autoboxing
Autoboxing is the automatic conversion that the Java compiler makes between the primitive types and their corresponding object wrapper classes. For example, converting an int to an Integer, a double to a Double, and so on.
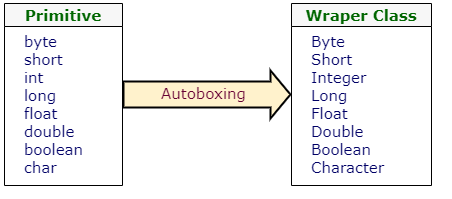
So, let’s use autoboxing in our code –
int val = 10;
List<Integer> intList = new ArrayList<Integer>();
intList.add(val);
Integer intObj = intList.get(0);
int output = intObj.intValue();
You can see, we did not convert the int value to integer object. It will be taken care of by the compiler.
Unboxing
Unboxing is the automatic conversion that the Java compiler makes from wrapper classes to corresponding primitive types. For example, converting an Integer to an int, a Double to a double, and so on.
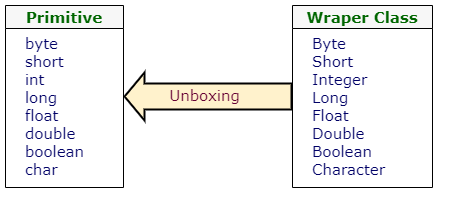
So, let’s rewrite the final version using unboxing –
int val = 10;
List<Integer> intList = new ArrayList<Integer>();
intList.add(val);
int output = intList.get(0);
So, you can see, using autoboxing and unboxing –
- We can write cleaner code
- We can write code that is easier to read and understand.
Autoboxing and unboxing with argument and return type
Autoboxing and unboxing can be applied everywhere including method arguments and return types.
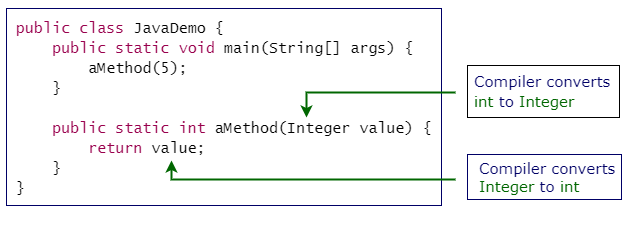
That’s it for now. Hope the concept of autoboxing, unboxing and wrapper classes is clear now. If you have any doubt, please ask in the comment section. I will try to answer that as soon as possible. Till then, bye bye.