Introduction
Java collection represents a group of individual objects that behaves as a single unit. The Java Collections Framework provides a set of interfaces and classes to create those groups. In one situation, you might want a group of objects in a sequential order because their ordering is important. In another situation, you might need a group where duplicates will be discarded. The Java Collections Framework will help you to deal with those situations. All of the classes and interfaces of the collection framework are bundled into the java.util
package.
Hierarchy of Collection Framework
Let us see the hierarchy of the Collection framework. We have intentionally removed a few classes to make it simpler and to highlight the mostly used classes.
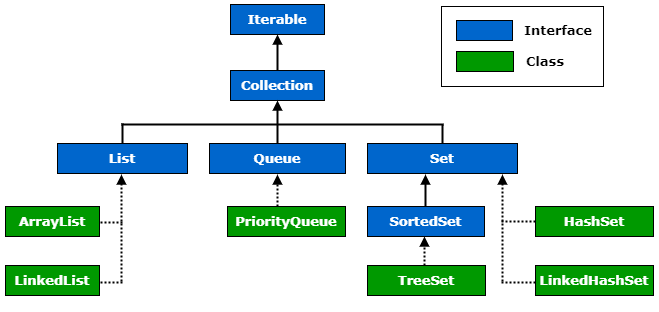
We will only discuss the interfaces
here and discuss the individual classes in a dedicated chapter.
Iterable
Iterable defines the contract that a class has to fulfill to be usable with the foreach
statement. We have seen the foreach
loop earlier. Foreach loop works with all the collection types. So, there must be some uniform way to access collection elements irrespective of the collection type. By implementing the Iterable
interface, all the collection classes agree to that uniform way.
This is the simplified version of the Iterable
interface –
public interface Iterable<T> {
Iterator<T> iterator();
}
So when a class implements the Iterable
interface, that class agrees to return an object of type Iterator
by overriding the iterator()
method.
This is the simplified version of Iterator
interface –
public Iterator<E> {
boolean hasNext();
E next();
void remove();
}
Let’s understand the purpose of individual methods –
- hasNext() : Is next element present.
- next() : Return the next element
- remove() : Remove the last element returned.
We have seen the foreach
loop earlier. Foreach loop makes use of those methods to loop through the collection.
List<String> list = Arrays.asList("I", "Love", "Java");
for (String element: list) {
System.out.print(element + " ");
}
Output: I Love Java
This is equivalent to the below code because foreach
loop is a concise way to use the Iterator
under the hood.
List<String> list = Arrays.asList("I", "Love", "Java");
for (Iterator<String> itr = list.iterator(); itr.hasNext(); ) {
String element = itr.next();
System.out.print(element + " ");
}
Output: I Love Java
Collection
The Collection interface defines the core functionality that we expect out of any collection like –
- Add an element.
- Remove an element.
- Check if an element is present in the collection.
- Get the size of the collection.
- Check if the collection is empty.
There are more. These are a few methods that are mostly used.
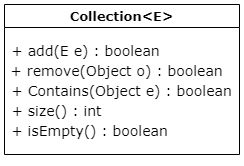
List
List is probably the most widely used Java collection. A list is a collection of ordered elements. Do not get confused between ordered and sorted. Ordered means the collection will maintain the sequence in which an element is added to the collection. Sorted means the elements are sorted based on sort criteria.
Let’s assume, you are adding four values in the following order: Banana, Apple, Mango, Cherry
.
- A sorted collection will show:
[Apple, Banana, Cherry, Mango]
- But an ordered collection like list will show:
[Banana, Apple, Mango, Cherry]
As the order is maintained, along with basic collection methods, you can add, remove or remove elements based on index. If you don’t specify the index during addition, an element will be added to the end.
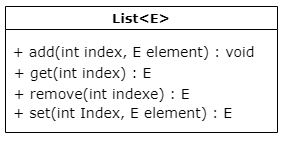
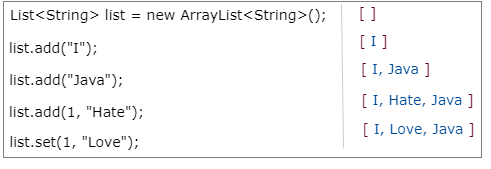
Queue
The purpose of a queue
is to hold elements for processing. In which order those elements will be processed is based on a rule. The order can be in which they are added (First In First Out, or FIFO) or based on priority etc.
Below are few of the mostly used Queue interface methods –
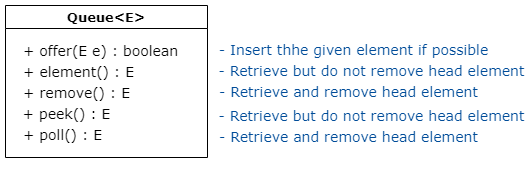
The remove()
and poll()
methods may look similar, but they differ only when the queue is empty. In case of an empty queue –
remove()
throwsNoSuchElementException
poll()
returnsnull
.
Similarly, element()
and peek()
methods may look similar, but they differ only when the queue is empty. In case of an empty queue –
element()
throwsNoSuchElementException
peek()
returnsnull
.
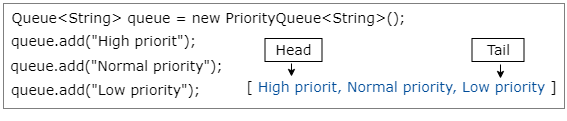
Set
A set is a collection that does not contain duplicate elements. If you try to add an element that is already present in the set, that element will be ignored.
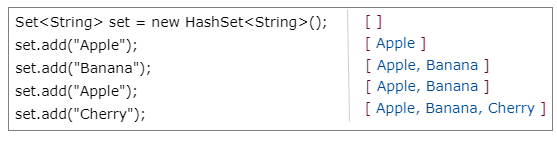
Map
There is another collection called Map that doesn’t come under the collection hierarchy. While other collections store only values, map stores key-value pairs. To add a key-value pair we can use the put()
method and to get a value by key, we can use get()
method.
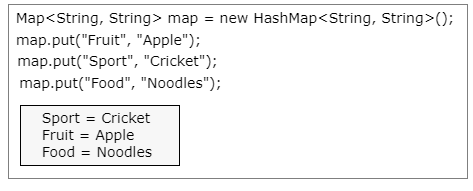
That’s it for now. Hope you have got the basic idea of the collection framework. We will discuss individual classes in detail in a dedicated chapter. If you have any doubt, please ask in the comment section. I will try to answer that as soon as possible. Till then, bye bye.