The basic purpose of java packages is to group related classes. To group files in your computer, you might create a folder pictures
to keep all image files and another folder videos
to store your favorite movies and so on. The main reason behind this is – it will help you to easily search one item. For example, if you want to play a movie, you will directly go to the videos
folder and play the movie of your choice. No need to search inside pictures
or any other folder. In java, you can consider packages
like those folders.
How to create a package and a class inside it?
Let’s consider we want to create a class HelloJava
inside package com.demo
.
For the rest of the courses we will use Eclipse IDE to write java codes. Eclipse or any other IDE (integrated development environment) help us to write, run and debug java codes more easily. So, download Eclipse now if not done yet.
- First of all, create a folder
java_workspace
underC:
drive and use this folder as Eclipse workspace. - In Eclipse, navigate to
File > New > Project… > Java Project
and click onNext
and enter the Project name asTestJava
. Then click onFinish
. You will see a folderTestJava
is created under thejava_workspace
folder. - In Eclipse, right click on the project name (i.e. TestJava) and navigate to
New > Package
. Enter package name ascom.demo
and click onFinish
. - Right click on the package name and navigate to
New > Class
and create a class by nameHelloJava
and put below content. If you run this class using the Run button in Eclipse toolbar, you will see output :Helo Java
.
package com.demo;
public class HelloJava {
public static void main(String[] args) {
System.out.println("Hello Java");
}
}
If you open the folder C:\java_workspace\TestJava
, you will see one src
folder, which will store all the source files that you will write. Under this src
folder, sub folders are created based on the package name.
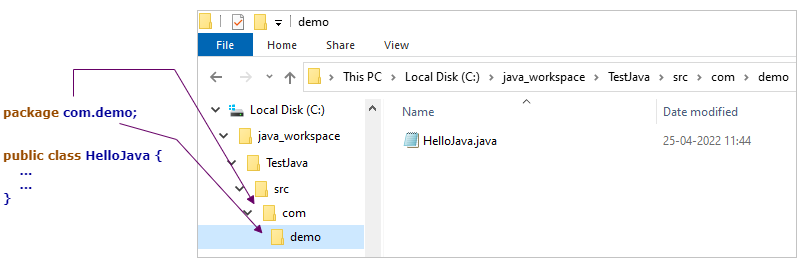
You see, as the package name is com.demo
, a folder called com
is created under the src
folder and under that com
folder, another folder demo
is created. And under this demo
folder, we have the java file HelloJava.java
.
Why do we use packages?
There are multiple reasons –
- Easy to Search: As packages group classes, it helps us to easily locate classes. For example we might have a package called
com.demo.util
to keep all utility classes. So, if we want to check what utility classes we already have, we can simply navigate to that package and check. - Preventing naming conflicts: If we want to create multiple classes with the same name, we can achieve that using packages. All we have to do is keep those classes under different packages
- Access Control: We know that default and protected access modifiers have package level access control as shown below table. So, packages along with access modifiers can help us to restrict access among different classes
Visibility | default | protected |
---|---|---|
Same class | Yes | Yes |
Non subclass in same package | Yes | Yes |
Subclass in same package | Yes | Yes |
Subclass in different package | No | Yes |
Non subclass in different package | No | No |
Package naming convention
- Package names are written in all lower case.
- Package names for in-built java classes begin with java. or javax.
- For the packages that we create for our application, normally we begin the package name with top level domain names like
com, gov, org
etc. Then thecompany name
. Then your own naming conventions. For example, if you are writing an utility class for companyABC
, you can put that class under package:com.abc.util
Types of packages
Packages can be divided into two categories –
- Built-in Packages: These are the packages from the Java API. For example, we have used
System.out.println()
to print a value. ThisSystem
is a class and present inside the built-in packagejava.lang
. Other commonly used built-in packages are –java.io, java.util
etc. - User-defined Packages: These are the packages that are created by us. For example, we have created a
com.demo
package to store classHelloJava.java
.
How to use classes from different packages?
To use a class from a different package, you need to use the import
keyword. If the class is present in the same package, no need to import anything. You can directly use that class.
To understand it better, let’s create two classes – ClassA
and ClassB
under package com.demo.pkg1
and another class ClassC
under package com.demo.pkg2
.
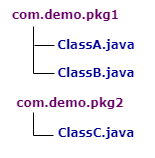
package com.demo.pkg1;
import com.demo.pkg2.ClassC;
public class ClassA {
public void sayHello() {
System.out.println("Hello");
}
public void hi() {
ClassB b = new ClassB();
b.sayHi();
}
public void bye() {
ClassC c = new ClassC();
c.sayBye();
}
}
package com.demo.pkg1;
public class ClassB {
public void sayHi() {
System.out.println("Hi");
}
}
package com.demo.pkg2;
public class ClassC {
public void sayBye() {
System.out.println("Bye");
}
}
If you look closely at ClassA
, you will see, to use ClassB
inside method hi()
, we didn’t import the class, as ClassB
belongs to the same package as ClassA
. But to use ClassC
inside method bye()
, we have imported the class as ClassC
belongs to a separate package.
We can import a single class or all classes from a package.
- To import a single class, use import packageName.ClassName
import com.demo.pkg2.ClassC
- To import all classes from a package, use import packageName.*
import com.demo.pkg2.*
Why don’t we import System class or String class?
The question is, as I said earlier, if we want to use a class from a separate package, we have to import that class. But we never import String
class or System
class. Let’s understand the question with below example –
public class TestJava {
public static void main(String[] args) {
String color = "Red";
System.out.println("My Favorite color is: " + color);
}
}
We have used both System
class and String
class in the example above. But we didn’t import any of them. Then how is it working?
The reason is, whenever you write a class, all classes from the java.lang
package are auto-imported as if the declaration import java.lang.*
is added automatically. Both System
and String
classes belong to the java.lang
package. So, explicit import is not required. Not only those two classes, if you want to use any classes from package java.lang
(e.g. Math, Thread
etc.), no need to import them.
If you import the String
class or any other class from the java.lang
package (e.g. import java.lang.String;
), this will compile fine, but that is just not necessary.
Class Name Conflict
Let’s consider the Date
class provided by java. Both java.util
and java.sql
package provide Date
class. So, if you try to import both of them, that will throw a compilation error.
import java.util.Date;
import java.sql.Date;
public class TestJava {}
Compilation Error at line 2: The import java.sql.Date collides with another import statement
.
But if you need both the classes in your class, what will you do? You can do something like below –
import java.util.Date;
import java.sql.*;
public class TestJava {
public static void main(String[] args) {
Date date1 = new Date();
System.out.println(date1);
}
}
Here date1
is an instance of explicitly declared java.util.Date
class. To create an instance of java.sql.Date
class, you have to specify fully qualified class name (class name along with package name) –
import java.util.Date;
import java.sql.*;
public class TestJava {
public static void main(String[] args) {
java.sql.Date date = new java.sql.Date(123);
System.out.println(date);
}
}
That’s it for now. Hope the concept of the package is clear now. We will meet again with a new topic. Till then bye bye.