In programming languages, loops are used to repeat a block of code multiple times as long as some condition is satisfied.
For example, let’s consider you have a list of 5 songs and you want to play them all. To achieve that, you may write a play()
method to play a song and you can call that method 5 times.
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("Song1", "Song2", "Song3", "Song4", "Song5");
play(songs.get(0));
play(songs.get(1));
play(songs.get(2));
play(songs.get(3));
play(songs.get(4));
}
private static void play(String song) {
System.out.println("Playing song : " + song);
}
}
Playing song : Song1
Playing song : Song2
Playing song : Song3
Playing song : Song4
Playing song : Song5
List<String> songs = Arrays.asList("Song1", "Song2");
Here “songs” is a list that can contain only String values and current values are “Song1” and “Song2”.
Our PlayAudio
class is doing the job perfectly. But, what if we have a thousand songs? Will you really be happy to repeat the same line a thousand times? Probably not right. Probably you will use something that will call the play()
method repeatedly until all the songs are finished. This is exactly what java loop will do.
There are three types of loops in Java –
- for loop
- while loop
- do-while loop
1. for loop
Let’s rewrite the PlayAudio class using for loop.
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("Song1", "Song2", "Song3", "Song4", "Song5");
for (int i=0; i<songs.size(); i++) {
play(songs.get(i));
}
}
private static void play(String song) {
System.out.println("Playing song : " + song);
}
}
Playing song : Song1
Playing song : Song2
Playing song : Song3
Playing song : Song4
Playing song : Song5
This is much better than the earlier version right? If you look carefully, there are four parts of a for
loop –
for (initialization; condition; increment or decrement) {
Code block
}
- Initialization: This is executed (only once) before the execution of the code block.
- Condition: This must return a boolean value (true or false). If this value is true, the code block will be executed.
- Increment or Decrement: It increments or decrements the initialized variable value.
- Code block: Statements to be executed for each iteration.
Flow Diagram:
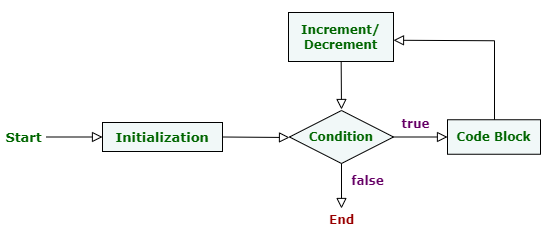
- First the initialization is executed. In our example, an
int
variablei
is created and the value0
is assigned to it. - Then the condition is tested. If the condition is true, execute the code block inside the loop body, otherwise exit the loop.
- Once the code block is executed, increment or decrement the initialized value. In our example, the value of
i
will be increased to1
from0
. - Then again the condition is tested (like step 2) and goes on repeatedly until the condition is false.
Enhance for loop or for-each loop
This is a special kind of for
loop which makes it easier to iterate over an array or any collection (like List
). for each
loop simply walk through all the elements one by one and execute the code block. Once there is no more element, the loop will terminate.
If we rewrite our code using for eac
h loop, it will look like below –
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("Song1", "Song2", "Song3", "Song4", "Song5");
for (String song : songs) {
play(song);
}
}
private static void play(String song) {
System.out.println("Playing song : " + song);
}
}
Playing song : Song1
Playing song : Song2
Playing song : Song3
Playing song : Song4
Playing song : Song5
2. while loop
Unlike for
loop, a while
loop contains only the condition. It doesn’t have built-in initialization or increment/decrement part. It looks similar to if
block the only difference is it repeats itself.
Syntax:
while (condition) {
Code block
}
Flow Diagram:
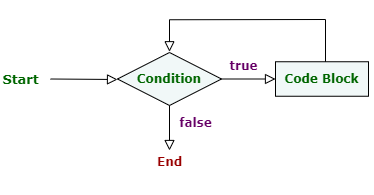
Example:
Let’s rewrite our code using while loop –
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("Song1", "Song2", "Song3", "Song4", "Song5");
int i=0;
while(i<songs.size()) {
play(songs.get(i));
i++;
}
}
private static void play(String song) {
System.out.println("Playing song : " + song);
}
}
Playing song : Song1
Playing song : Song2
Playing song : Song3
Playing song : Song4
Playing song : Song5
3. Do while loop
do while
loop is similar to while
loop with only difference that it checks for condition after executing the code block. So, if the condition is false for the very first time, for a while
loop, code block will not be executed. But in the case of do while
loop, code block will be executed once as the condition is checked after executing the code block.
Syntax:
do {
Code block
}
while (condition);
Flow Diagram:
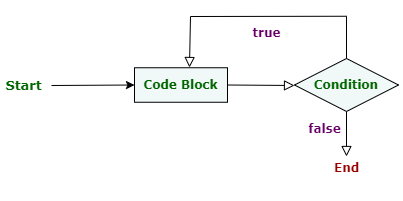
Example:
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("Song1", "Song2", "Song3", "Song4", "Song5");
int i=0;
do {
play(songs.get(i));
i++;
} while(i<songs.size());
}
private static void play(String song) {
System.out.println("Playing song : " + song);
}
}
Continue and break
Break : We have already seen the break
statement in the switch
statement. The break
statement will perform exactly the same operation – it will tell the JVM to come out of the loop.
Let’s assume we have a list of songs and we want to check if any song starts with “la”. To do that, we will iterate over all the elements of the list and check one by one if any song starts with the word “la”. But if a match is found, is it necessary to check further? No, right. So, we can simply use the break statement to come out of the loop once a match is found.
Example:
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("he he", "la la", "ho ho");
boolean isMatched = false;
for (String song : songs) {
if (song.startsWith("la")) {
isMatched = true;
break;
}
}
System.out.println("Match found : " + isMatched);
}
}
Match found : true
Please remember, it will only exit the current loop. If this loop is inside another loop, it will not exit the outer loop.
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("he he", "la la", "ho ho");
for (int i=0; i<3; i++) {
System.out.println("value of i : " + i);
for (String song : songs) {
System.out.println(song);
if (song.startsWith("la")) {
break;
}
}
}
}
}
value of i : 0
he he
la la
value of i : 1
he he
la la
value of i : 2
he he
la la
Continue: The continue statement breaks only one iteration if a specified condition is true, and continues with the next iteration in the loop.
Let’s assume we have a list of songs and we want to print song names only if the song name doesn’t start with “la”. To do that, we can use a continue statement to skip printing the name based on the condition.
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("he he", "la la", "ho ho");
for (String song : songs) {
if (song.startsWith("la")) {
continue;
}
System.out.println(song);
}
}
}
he he
ho ho
Infinite loop
An infinite loop endlessly executes the loop body as the terminating condition never met. Let’s consider below example –
public class PlayAudio {
public static void main(String[] args) {
List<String> songs = Arrays.asList("he he", "la la", "ho ho");
for (int i=0; i<songs.size(); ) {
System.out.println(songs.get(i));
}
}
}
As we have not specified the increment/decrement part, the value of i
will always remain 0
and this code will endlessly print “he he”.
Most of the time the infinite loop occurs due to programming error, but may also be intentional for special use cases.
I hope the concept of loop is pretty clear now. We will meet again with a new topic. Bye bye.