String
In Java, String is basically an object that represents a sequence of char
values. In other words Strings are objects for storing text values.
How can we create a String object?
There are two ways –
Method | Example |
---|---|
By String literal | String s = “Hello World”; |
By new keyword | String s = new String(“Hello World”); |
Generally when we want to create an instance of a class, we use the new
keyword. This new
keyword basically creates a new object in heap memory and returns a reference to that memory. This is exactly what we did in the second method.
If we want to use the first method (the most common way), we have to pass a text value inside a double quote and it will return a String object.
Both methods seem like doing the same thing, right? Not exactly. Before going into detail, let’s first understand String Constant Pool (SCP)
.
String Constant Pool
String Constant Pool or String Pool is a separate place in the heap memory where the values of all the strings are stored.
When we create any object using the new
keyword, a new object gets created in the heap memory. Those objects are removed by the Garbage Collector from the heap when they are not in use.
A normal Java application deals with thousands of string objects and lots of them have the same value. So if we store all those string objects in normal heap memory, a lot of the heap will be acquired by just String objects only. And the worst part is, many of those objects will hold identical values. So, to make heap space available, Garbage Collector will have to run more frequently which in turn will decrease the performance of the application.
That’s why we have the String Constant Pool to store unique string values.
Difference between creating a String using string literal and new keyword
Let’s understand the string object creation using literal with an example.
String str1 = "Hello";
String str2 = "Hello";
String str3 = "World";
System.out.println(str1 == str2);
- For the first line, JVM checks if that string already exists in the string constant pool. Initially it won’t find any. So, a new string instance is created and placed in the pool. And the reference is returned and assigned to the
str1
variable. - For the second line, JVM again checks for the string in the pool. This time JVM will find it already exists. So, a new instance will not be created, simply the reference to the pooled instance is returned and that reference is assigned to the
str2
variable. - For the third line, JVM won’t find any matching string in the pool. So, a new string instance is created and placed in the pool. And the reference is returned and assigned to the
str3
variable. - As both
str1
andstr2
are referring to the same object, it will returntrue
.
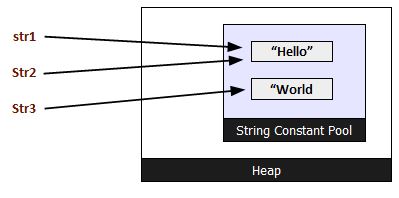
Now consider doing the same thing using the new keyword.
String str1 = new String("Hello");
String str2 = new String("Hello");
String str3 = "Hello";
String str4 = new String("World");
System.out.println(str1 == str2);
- First line will create a new string object in the heap with the value “Hello”. Also it will add a new instance in the pool.
- Second line will again create a new string object in the heap with the value “Hello”. But this time, as the value is already present in the pool, no instance will be added to the pool.
- Third line will return the existing pooled instance as it already exists. Nothing will be created in the pool or heap.
- Fourth line will create a new string object in the heap with the value “World”. Also it will add a new instance in the pool as this doesn’t exist.
- Fifth line will return
false
as although the value is the same (“Hello”), both are referring to different objects in the heap.
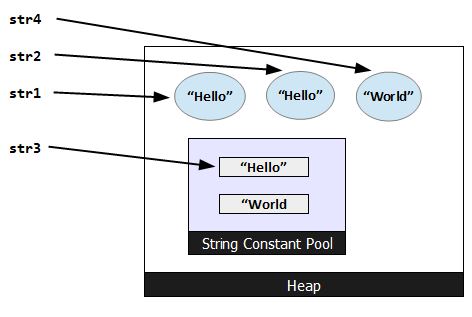
Basic String operations
Operation | Example | Output |
---|---|---|
Get string length | String str1 = "Hello World"; | 11 |
Finding the index of a text inside another string (index starts from 0) | String str1 = "Hello World"; | 6 |
Check if a string contains another string | String str1 = "Hello World"; | true |
Concat multiple strings | String str1 = "Hello " + "World"; | Hello World |
String is immutable
One point I must mention is, String class is immutable. Immutable means, once an object is created, the state of that object cannot be changed. That means, once an object of string is created, its value can not be altered. Now let’s examine the code below.
String str1 = "Hello";
str1 = "World";
You might think, wait a minute – here initially the value of str1
was “Hello”. Then we have changed the value to “World”. How is it immutable then?
The answer is, initially when you have created a string with value “Hello”, a new instance is created in String Pool. The reference of that instance is assigned to variable str1
. Now when you have re-assigned a new value “World”, internally again a new instance is created for “World” in the pool and that reference is assigned to str1
. So you see, even if the reference got changed, the actual “Hello” instance is not modified, rather a new instance is created in the pool and reference of that instance is re-assigned.
Math
The Java Math class provides many methods that allow us to perform mathematical tasks on numeric values.
Basic Math operations
Operation | Example | Output |
---|---|---|
Get highest value between two numbers | Math.max(3, 4.5) | 4.5 |
Get lowest value between two numbers | Math.min(3, 4.5) | 3.0 |
Get square root of a number | Math.sqrt(16) | 4.0 |
Get power of a number | Math.pow(2, 3) | 8.0 |
Get absolute value (positive) of a number | Math.abs(-3.24) | 3.24 |
Get closest whole number | Math.round(3.24) | 3 |
Get the smallest whole number that is greater than or equal to the argument. | Math.ceil(3.24) | 4.0 |
Get the highest whole number that is less than or equal to the argument. | Math.floor(3.24) | 3.0 |
Get a random between 0.0 (inclusive), and 1.0 (exclusive) | Math.random() | 0 <= value < 1 |
Get a random number between 0 (inclusive), and 20 (inclusive) | (int)(Math.random() * 21) | 0 <= value <= 20 |
I hope the concept of String and Math class is clear now. We will meet again with a new topic.